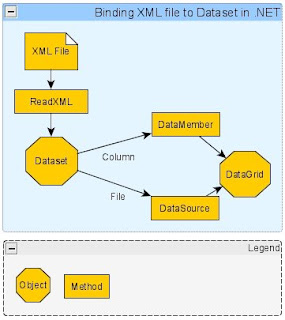
I've been exploring the various ways that .NET offers to parse XML files. Luckily it seems like the more I learn, the simpler ways I discover to do a small task. This small task is to read an XML file and show it in a DataGrid. I found it in this
website .
Putting it all together
You will need to add an XML file into your build. For this sample, this is the XML file. <?xml version="1.0" encoding="utf-8" ?><family> <name gender="Male"> <firstname>Tom</firstname> <lastname>Smith</lastname> </name> <name gender="Female"> <firstname>Dale</firstname> <lastname>Smith</lastname> </name></family>
In a new VB Windows form, add a DataGrid, call it grdData. Add a button to handle your event, and put this code:
Imports System
Imports System.Data
Imports System.Data.SqlClient
Public Class ReadXMLtoDataset
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Try
Dim dsPubs As New DataSet()
' Read in XML from file
dsPubs.ReadXml("C:\mySandbox\vbSandbox2\vbSandbox2\scoreTableXML\myScores.xml")
' Bind DataSet to Data Grid
grdData.DataMember = "name"
grdData.DataSource = dsPubs
Catch ex As Exception
MessageBox.Show(ex.Message)
End Try
End Sub
End Class
This is a simple way of putting together a quick bind to an XML file.